Wall Maker in Maya with Python
- anderson Yang
- Feb 13, 2023
- 5 min read
A Python script for create brick wall. This script incluides two options to choose, one is cross arrangement, the other one is without arrangement. Users can also adjust how many bricks of row and column, the minimum/maximun is 1 and 9999. There are 3 different bricks that users can choose, solid bricks, Two-holes Hollow bricks, and Three-holes Hollow bricks. And the wall is RBD ready.

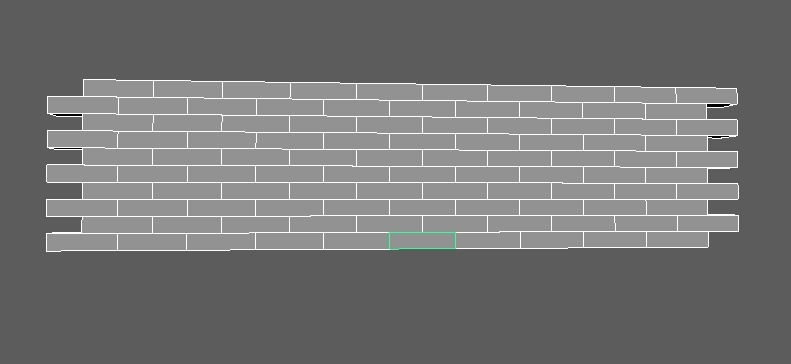
Python Module
"""Script for Maya
Version 01
Author: Anderson Yang
Date: 02/12/23
Contacts:
-Instagram: yang_vfx
-Mail: besthans0127@gmail.com
-LinkedIn: https://www.linkedin.com/in/hanyang0127/
Description: This code will generate bricks and wall, and if selecting the arrangement option, it can also generate cross structure wall.
Tested on Maya 2022.3
"""
import maya.cmds as mc
import math
# Window setting
WallMaker = mc.window(title = "Wall Maker", wh = (500, 275))
mc.rowColumnLayout(adjustableColumn = True, backgroundColor = (0.3, 0.3, 0.3))
# Close the tool
def exit():
mc.deleteUI(windows, window = True)
# Triangulate
def polyTri():
sel = mc.ls(selection = True)
for i in sel:
mc.polyTriangulate(i)
# Quadrangulate
def polyQua():
sel = mc.ls(selection = True)
for i in sel:
mc.polyQuad(i)
# A window shows the information of this script
def aboutInfo():
aboutInformation = mc.window(title = "Wall Maker for Maya by Anderson Yang", wh = (512, 200))
mc.rowColumnLayout(adjustableColumn = True, backgroundColor = (0.15, 0.15, 0.15))
mc.text(label = "Wall Maker for Maya 1.0 © 2023 Anderson Yang 02-13-2023")
mc.separator(height = 50, style = "out")
mc.text(label = "This is the first release of the Wall Maker for Maya.\n Please report any bugs or requests, and stay tuned for the full release.")
mc.separator(height = 50, style = "out")
mc.text(label = "Feel free to contact me directly about any issue at besthans0127@gmail.com \n ")
mc.showWindow(aboutInformation)
# Open google sheet for bugs report
def bugsReport():
mc.showHelp("https://forms.gle/xLMFcqLyyB1rmEaK6", absolute = True)
def applyWall():
createWall()
# Menu bar layout
firstMBL = mc.menuBarLayout()
mc.menu(label = "Edit")
mc.menuItem(label = "Triangulate", command = "polyTri()")
mc.menuItem(label = "Quadrangulate", command = "polyQua()")
mc.menu(label = "Help", helpMenu = True)
mc.menuItem(label = "Report Bugs", command = "bugsReport()")
mc.menuItem(label = "About", command = "aboutInfo()")
# Frame layout
layout = mc.columnLayout(adjustableColumn = True)
# Brick framlayout
brickFrame = mc.frameLayout(collapsable = True, label = "Brick", width = 100)
subFrameBrick = mc.radioCollection()
mc.columnLayout()
brickType = mc.radioButtonGrp(label = "Style", labelArray3 = ["Solid", "Two Holes", "Three Holes"], numberOfRadioButtons = 3, cal = [1, "center"], onc = "createWall()")
mc.setParent("..")
mc.setParent("..")
# Mansonry Wall framlayout
mansonryFrame = mc.frameLayout(collapsable = True, label = "Mansonry Wall")
mansonryCheck = mc.radioButtonGrp(label = "", labelArray2 = ["No Arrangement", "Cross Arrangement"], numberOfRadioButtons = 2, cal = [1, "center"])
mc.setParent("..")
mc.setParent("..")
# Order framlayout
wallFrame = mc.frameLayout(collapsable = True, label = "Row & Column")
row = mc.intSliderGrp(field=True, label = "Row", minValue = 0, maxValue = 10, fieldMinValue = 0, fieldMaxValue = 9999, value = 0, cal = [1, "center"] )
column = mc.intSliderGrp(field=True, label = "Column", minValue = 0, maxValue = 10, fieldMinValue = 0, fieldMaxValue = 9999, value = 0, cal = [1, "center"] )
mc.setParent("..")
mc.setParent("..")
# Wall generate Pattern
def createWall(*args):
# grab the value from options
columnValue = mc.intSliderGrp(column, query = True, value = True)
rowValue = mc.intSliderGrp(row, query = True, value = True)
pattern = mc.radioButtonGrp(brickType, query = True, select = True)
mansonryPattern = mc.radioButtonGrp(mansonryCheck, query = True, select = True)
# Solid bricks and no mansonry
if (pattern == 1 and mansonryPattern == 1):
for r in range(0, rowValue):
mc.polyCube(w = 2, h = 0.5, d = 1, name = "Brick" + str(r))
mc.move(2 * (r), 0, 0)
mc.select(all = True)
mc.group(name = "G1")
for c in range(1, columnValue):
mc.duplicate(name = "G" + "_" + str(c))
mc.move(0, (0.5 * c), 0, "G_" + str(c))
mc.select(all = True)
mc.ungroup()
mc.delete(constructionHistory = True)
# Two holes bricks and no mansonry
elif (pattern == 2 and mansonryPattern == 1):
cy = mc.polyCylinder(r = 0.5)
mc.select(cy)
mc.move(-1, 0, 0)
cy1 = mc.polyCylinder(r = 0.5)
mc.select(cy1)
mc.move(1, 0, 0)
mc.select(all = True)
mc.polyBoolOp("pCylinder1", "pCylinder2", op = 1)
mc.delete(constructionHistory = True)
mc.polyCube(w = 4, h = 1, d = 2)
mc.polyBoolOp("pCube1", "polySurface1", op = 2)
mc.select("polySurface2")
mc.delete(constructionHistory = True)
mc.rename("polySurface2", "box")
for r in range(0, rowValue):
mc.duplicate(name = "Brick" + "_" + str(r))
mc.move((4*r), 0, 0)
mc.delete("box")
mc.select(all = True)
mc.group(name = "G1")
for c in range(1, columnValue):
mc.duplicate(name = "G" + "_" + str(c))
mc.move(0, (c), 0, "G_" + str(c))
mc.select(all = True)
mc.ungroup()
# Three holes bricks and no mansonry
elif (pattern == 3 and mansonryPattern == 1):
c3 = mc.polyCylinder(r = 0.5)
mc.select(c3)
mc.move(-1.3, 0, 0)
c31 = mc.polyCylinder(r = 0.5)
c32 = mc.polyCylinder(r = 0.5)
mc.select(c32)
mc.move(1.3, 0, 0)
mc.select(all = True)
mc.polyBoolOp("pCylinder1", "pCylinder2", op = 1)
mc.polyBoolOp("pCylinder3", "polySurface1", op = 1)
mc.delete(constructionHistory = True)
mc.polyCube(w = 4, h = 1, d = 2)
mc.polyBoolOp("pCube1", "polySurface2", op = 2)
mc.select("polySurface3")
mc.delete(constructionHistory = True)
mc.rename("polySurface3", "box")
for r in range(0, rowValue):
mc.duplicate(name = "Brick" + "_" + str(r))
mc.move((4*r), 0, 0)
mc.delete("box")
mc.select(all = True)
mc.group(name = "G1")
for c in range(1, columnValue):
mc.duplicate(name = "G" + "_" + str(c))
mc.move(0, (c), 0, "G_" + str(c))
mc.select(all = True)
mc.ungroup()
# Solid bricks and mansonry
elif (pattern == 1 and mansonryPattern == 2):
for m in range(0, rowValue):
mc.polyCube(w = 2, h = 0.5, d = 1, name = "Brick" + str(m))
mc.move(2 * (m), 0, 0)
mc.select(all = True)
mc.group(name = "G1")
for s in range(1, 2):
mc.duplicate(name = "G" + "_" + str(s))
mc.move(1, (0.5 * s), 0, "G_" + str(s))
mc.select(all = True)
mc.ungroup()
mc.group(name = "G2")
if (columnValue % 2 == 0):
for n in range(1, int(abs(columnValue*(0.5)))):
mc.duplicate(name = "G" + "_" + str(n))
mc.move(-0.001, n, 0, "G_" + str(n))
mc.select(all = True)
mc.ungroup()
if (columnValue % 2 == 1):
for n in range(1, int(abs(columnValue * 0.5))+1):
mc.duplicate(name = "G" + "_" + str(n))
mc.move(-0.001, n, 0, "G_" + str(n))
mc.ungroup()
lastLayer = mc.ls(selection = True)
print(lastLayer)
for i in range(rowValue, len(lastLayer)):
mc.delete(lastLayer[i])
mc.group(name = "G_")
mc.select(all = True)
mc.ungroup()
# Two holes bricks and mansonry
elif (pattern == 2 and mansonryPattern == 2):
cy = mc.polyCylinder(r = 0.5)
mc.select(cy)
mc.move(-1.3, 0, 0)
cy1 = mc.polyCylinder(r = 0.5)
mc.select(cy1)
mc.move(1.3, 0, 0)
mc.select(all = True)
mc.polyBoolOp("pCylinder1", "pCylinder2", op = 1)
mc.delete(constructionHistory = True)
mc.polyCube(w = 4, h = 1, d = 2)
mc.polyBoolOp("pCube1", "polySurface1", op = 2)
mc.select("polySurface2")
mc.delete(constructionHistory = True)
mc.rename("polySurface2", "box")
for r in range(0, rowValue):
mc.duplicate(name = "Brick" + "_" + str(r))
mc.move((4*r), 0, 0)
mc.delete("box")
mc.select(all = True)
mc.group(name = "G1")
for c in range(1, 2):
mc.duplicate(name = "G" + "_" + str(c))
mc.move(2, (c), 0, "G_" + str(c))
mc.select(all = True)
mc.group(name = "G2")
if (columnValue % 2 == 0):
for n in range(1, int(abs(columnValue*(0.5)))):
mc.duplicate(name = "Tw" + "_" + str(n))
mc.move(-0.001, 2*n, 0, "Tw_" + str(n))
mc.select(all = True)
mc.ungroup()
mc.ungroup()
if (columnValue % 2 == 1):
for n in range(1, int(abs(columnValue * 0.5))+1):
mc.duplicate(name = "Tw" + "_" + str(n))
mc.move(-0.001, 2*n, 0, "Tw_" + str(n))
mc.ungroup()
mc.ungroup()
lastLayer = mc.ls(selection = True)
print(lastLayer)
for i in range(rowValue, len(lastLayer)):
mc.delete(lastLayer[i])
mc.group(name = "Tw_")
mc.select(all = True)
mc.select("*Tw*", "*G*")
mc.ungroup()
# Three holes bricks and mansonry
elif (pattern == 3 and mansonryPattern == 2):
c3 = mc.polyCylinder(r = 0.5)
mc.select(c3)
mc.move(-1.3, 0, 0)
c31 = mc.polyCylinder(r = 0.5)
c32 = mc.polyCylinder(r = 0.5)
mc.select(c32)
mc.move(1.3, 0, 0)
mc.select(all = True)
mc.polyBoolOp("pCylinder1", "pCylinder2", op = 1)
mc.polyBoolOp("pCylinder3", "polySurface1", op = 1)
mc.delete(constructionHistory = True)
mc.polyCube(w = 4, h = 1, d = 2)
mc.polyBoolOp("pCube1", "polySurface2", op = 2)
mc.select("polySurface3")
mc.delete(constructionHistory = True)
mc.rename("polySurface3", "box")
for r in range(0, rowValue):
mc.duplicate(name = "Brick" + "_" + str(r))
mc.move((4*r), 0, 0)
mc.delete("box")
mc.select(all = True)
mc.group(name = "G1")
for c in range(1, 2):
mc.duplicate(name = "G" + "_" + str(c))
mc.move(2, (c), 0, "G_" + str(c))
mc.select(all = True)
mc.group(name = "G2")
if (columnValue % 2 == 0):
for n in range(1, int(abs(columnValue*(0.5)))):
mc.duplicate(name = "Tw" + "_" + str(n))
mc.move(-0.001, 2*n, 0, "Tw_" + str(n))
mc.select(all = True)
mc.ungroup()
mc.ungroup()
if (columnValue % 2 == 1):
for n in range(1, int(abs(columnValue * 0.5))+1):
mc.duplicate(name = "Tw" + "_" + str(n))
mc.move(-0.001, 2*n, 0, "Tw_" + str(n))
mc.ungroup()
mc.ungroup()
lastLayer = mc.ls(selection = True)
print(lastLayer)
for i in range(rowValue, len(lastLayer)):
mc.delete(lastLayer[i])
mc.group(name = "Tw_")
mc.select(all = True)
mc.select("*Tw*", "*G*")
mc.ungroup()
# Buttons for appling the options and cancel, close the window
mc.separator(height = 30, style = "none")
mc.button(label = "Apply", command = "applyWall()", backgroundColor = [0.4, 0.4, 0.4])
mc.separator(height = 10, style = "none")
mc.button(label = "Cancel", command = "exit()", backgroundColor = [0.4, 0.4, 0.4])
mc.showWindow(WallMaker)
Comments